Test e benchmark
Nei primi due articoli, abbiamo sviluppato, ottimizzato e profilato un algoritmo di sfocatura gaussiana basato su WebGPU. Grazie an un’interfaccia utente dedicata, è possibile osservare l’effetto applicato in tempo reale. Tuttavia, una semplice ispezione visiva non è sufficiente per rispondere a diverse domande:
- Il fragment shader calcola correttamente la convoluzione secondo il kernel gaussiano?
- La versione ottimizzata a due passate produce lo stesso risultato dell’implementazione standard?
- Lo shader si adatta correttamente a formati di texture differenti?
Una suite di test unitari può fornire risposte chiare a questi interrogativi, assicurando inoltre il corretto funzionamento del codice nel tempo, in caso di future evoluzioni. C’è però un problema: WebGPU non è supportato in ambiente Node.js, nemmeno con l’ausilio di librerie come jsdom, che simula solo parzialmente il comportamento del browser.
#Struttura del progetto
QUnit è un framework di test che può essere eseguito ovunque, incluso il browser in modo nativo. Questa caratteristica lo rende ideale per testare codice basato su WebGPU, visto che nel browser possiamo accedere senza limitazioni alle API necessarie.
Il progetto GitHub organizza il codice sorgente e i test in due cartelle separate,
src/
e test/
, seguendo una convenzione simile a quella comunemente adottata in Java.
src/ filter/ gauss_blur_2d.ts gauss_blur_2d_optimized.ts utils/ math.ts polynomial-regression.ts range.ts slice_matrix.ts webgpu/ generate_texture.ts read_texture.ts texture_metadata.ts timer.ts async_process_handler.ts main.ts vite-env.d.tstest/ browser/ filter/ gauss_blur_2d.qunit.ts utils/ texture_assert.ts webgpu/ read_texture.qunit.ts index.html main.qunit.ts qunit.css vite.config.ts vite-env.d.ts node/ utils/ math.spec.ts polynomial-regression.spec.ts range.spec.ts slice_matrix.spec.ts jest.config.js resources/ .gitkeep gauss_blur.py...index.htmlpackage.jsonstyle.csstsconfig.jsonvite.config.ts
La cartella dei test è organizzata in tre sottocartelle principali:
browser/
: include i test eseguiti nel browser utilizzando QUnit.node/
: contiene i test eseguiti in ambiente Node.js con Jest.resources/
: raccoglie dati esterni utilizzati come risorse dai test.
I file di test utilizzano un suffisso dedicato, come .qunit.ts
e .spec.ts
, e seguono una struttura speculare
alla cartella dei sorgenti. Ad esempio, il file test/browser/gauss_blur_2d.spec.ts
contiene i test per
src/gauss_blur_2d.ts
e src/gauss_blur_2d_optimized.ts
, poichè in questo caso conviene testare insieme le due funzionalità
strettamente correlate. Allo stesso modo, il file src/utils/slice_matrix.ts
è testato in node/utils/slice_matrix.spec.ts
.
Per semplificare la gestione, i file di configurazione di QUnit e Jest sono collocati all’interno delle rispettive cartelle, invece che nella root del progetto. In questo modo, ogni framework rimane autocontenuto e facilmente identificabile.
#Setup di QUnit
Per utilizzare QUnit, è necessario installare le dipendenze richieste. Con pnpm, ad esempio, il comando è il seguente:
pnpm add -D qunit @types/qunit
Il file test/browser/vite.config.ts
contiene la configurazione Vite necessaria per traspilare i test:
import { defineConfig } from 'vite';import path from "path";
export default defineConfig({ resolve: { alias: { '@': path.resolve(__dirname, '../../src'), '@test': path.resolve(__dirname, '../'), '@resources': path.resolve(__dirname, '../resources') } }, root: './test/browser', server: { port: 4000, open: false }, build: { outDir: 'dist/tests', emptyOutDir: true }});
La configurazione definisce un alias @
che punta alla cartella dei sorgenti, un alias @resources
per la cartella
delle risorse e un alias @test
che punta alla cartella dei test.
Il punto di ingresso predefinito per la compilazione è il file test/browser/index.html
:
<!DOCTYPE html><html lang="en"><head> <meta charset="UTF-8"> <title>QUnit Tests</title></head><body> <div id="qunit"></div> <div id="qunit-fixture"></div> <script type="module" src="./main.qunit.ts"></script></body></html>
Il div con l’id qunit
è usato dal framework per iniettare la sua interfaccia utente.
Il div con l’id qunit-fixture
, invece, può essere usato dai test per creare elementi nel DOM. È importante però tenere
a mente che questo div viene sempre svuotato tra un test e l’altro. Pertanto, non è adatto per visualizzare i risultati,
come ad esempio la texture ottenuta applicando la sfocatura gaussiana.
Il file test/browser/main.qunit.ts
include il framework QUnit e il relativo foglio di stile:
import { test } from "qunit";import './qunit.css';
test("QUnit works", assert => { // Expect 0 assertions in this test assert.expect(0);});
Il foglio di stile di QUnit può essere copiato dalla directory node_modules/
alla cartella dei test del browser con
il comando seguente:
cp node_modules/qunit/qunit/qunit.css test/browser
Per semplificare l’esecuzione dei test, nel file package.json
sono definiti degli script:
{ "scripts": { "test:node": "jest --config test/node/jest.config.js", "test:browser": "vite --config test/browser/vite.config.ts" }}
I test QUnit vengono eseguiti lanciando il comando npm run test:browser
, per poi visitare l’indirizzo http://localhost:4000/
nel
browser.
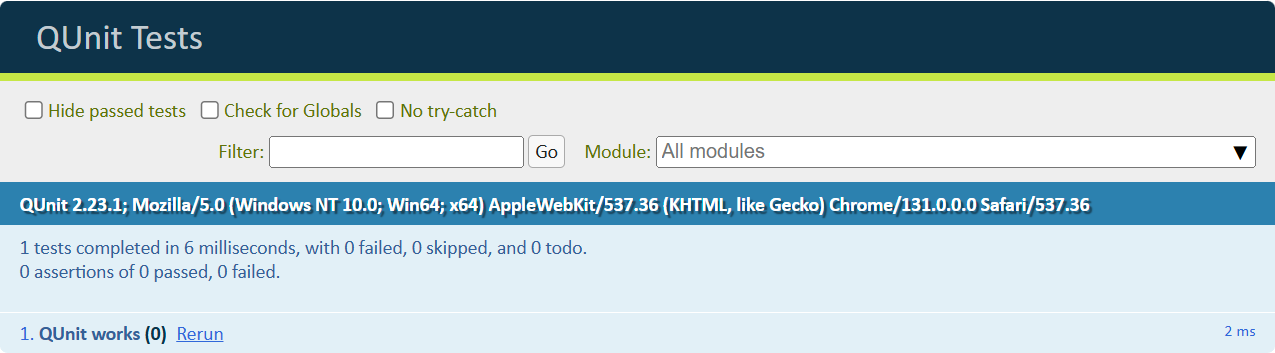
Interfaccia Web di QUnit
Con questi passaggi, il setup di QUnit è completo.
#Utilizzo di QUnit
In QUnit, ciascun test è definito dalla funzione test()
:
import { test } from 'qunit';
test("QUnit works", assert => { assert.expect(0);});
La funzione test
accetta due parametri: il nome del test e una funzione anonima che incapsula il test vero e proprio.
Il parametro assert
è un oggetto che fornisce diversi metodi per effettuare asserzioni. Nell’esempio il metodo expect()
serve a dichiarare il numero di asserzioni che si prevede vengano eseguite durante il test.
Test correlati tra loro possono essere raggruppati in moduli utilizzando la funzione module()
:
import { module } from 'qunit';
module("Math is not an opinion", hooks => { test("Basic algebra", assert => { assert.equal(2 + 2, 4, "sum works"); assert.equal(2 - 3, -1, "difference works"); });
test("Trigonometry", assert => { assert.equal(Math.cos(Math.PI), -1, "cosine works"); assert.true(Math.sin(Math.PI / 4) > 0, "sine works"); });});
I test possono fare uso di async/await
per gestire operazioni asincrone:
import { module } from 'qunit';
module("WebGPU", hooks => { test("is available", async assert => { assert.ok(navigator.gpu, "WebGPU is supported");
const adapter = await navigator.gpu.requestAdapter(); assert.ok(adapter, "Able to request GPU adapter"); });});
L’oggetto hooks
passato al modulo fornisce l’accesso al lifecycle dei test, permettendo di eseguire del codice in momenti
specifici prima e dopo l’esecuzione dei test:
module("Texture Read", hooks => { let adapter: GPUAdapter; let device: GPUDevice;
hooks.before(async (assert) => { // Request the GPU adapter adapter = (await navigator.gpu.requestAdapter())!; assert.ok(adapter, "Able to request GPU adapter");
// Request the GPU device device = await adapter.requestDevice(); assert.ok(device, "Able to request GPU device"); });
test("works with non padded texture", async (assert) => { // "device" can be used });
test("works with padded texture", async (assert) => { // "device" can be used also here });});
Oltre a before
, che viene eseguito prima di tutti i test, sono disponibili anche after
, beforeEach
e afterEach
per
gestire operazioni da eseguire rispettivamente dopo tutti i test, prima di ciascun test o dopo ciascun test.
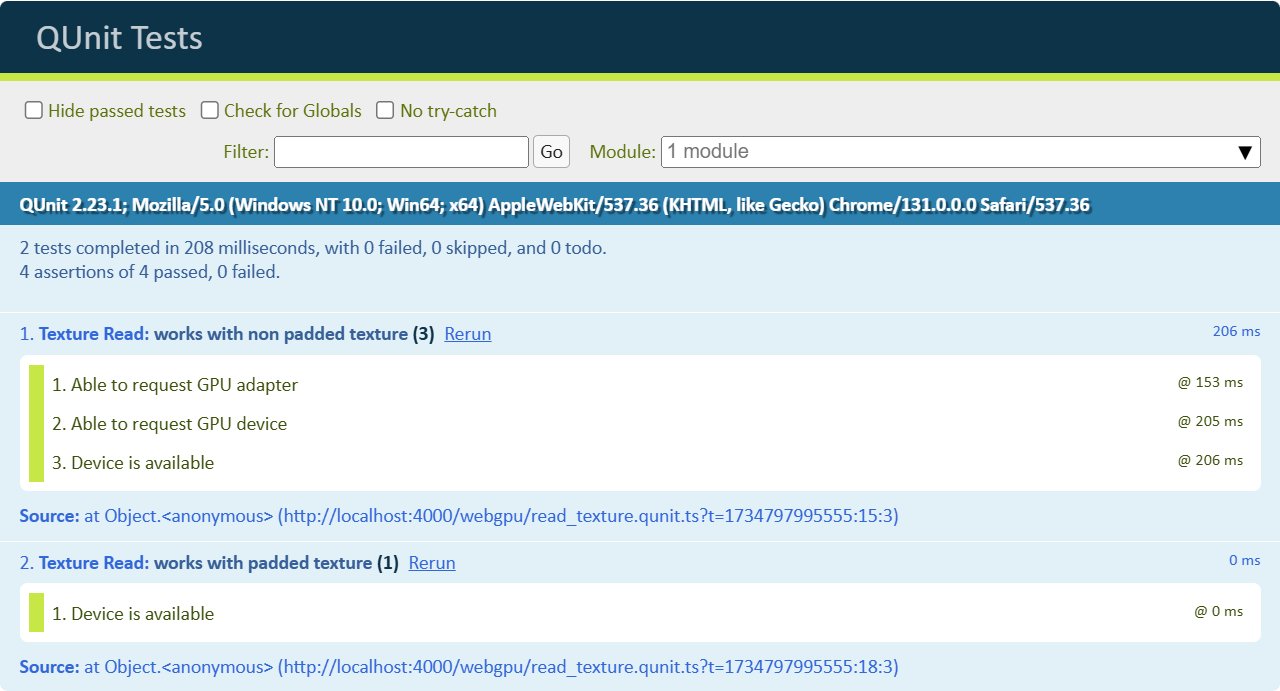
Le asserzioni contenute in before vengono eseguite una sola volta.
#Utility per le texture
I test per la sfocatura gaussiana hanno come obiettivi:
- Verificare il corretto funzionamento dell’implementazione di base;
- Verificare il corretto funzionamento dell’implementazione ottimizzata;
- Verificare il corretto funzionamento con formati diversi di texture;
Durante i test, sarà più volte necessario:
- Creare texture da da fornire come input.
- Trasferire le texture calcolate dalla GPU alla CPU.
- Comparare il contenuto delle texture con i valori attesi.
Poiché queste operazioni sono comuni e richiedono diversi passaggi, è opportuno creare dei metodi di utility per rendere i test più leggibili e facilitare la loro manutenzione.
#Generazione texture
La funzione generateTexture()
è dedicata alla generazione di una texture a partire da dati predefiniti:
import { TEXTURE_FORMAT_INFO, TypedArray } from '@/texture_metadata.ts';
export function generateTexture( device: GPUDevice, format: GPUTextureFormat, width: number, height: number, data: TypedArray, usage: GPUBufferUsageFlags, label: string): GPUTexture { const { bytesPerTexel } = TEXTURE_FORMAT_INFO[format]; const bytesPerRow = bytesPerTexel * width; const texture = device.createTexture({ size: { width, height }, format, usage: GPUTextureUsage.COPY_DST | usage, label });
device.queue.writeTexture( { texture }, data, { bytesPerRow, rowsPerImage: height }, [ width, height ] );
return texture;}
Questa funzione alloca la memoria con createTexture()
e poi trasferire il buffer data
verso la GPU utilizzando
writeTexture()
. I metadati di TEXTURE_FORMAT_INFO
forniscono il numero di byte per ciascun texel, necessario per calcolare
il numero totale di byte per riga richiesto da writeTexture()
.
Nei test, può essere utilizzata in questo modo:
test('Texture', assert => { const data = new Float32Array([ 1, 2, 3, 4, 5, 6, 7, 8, 9 ]);
let inputTexture: GPUTexture | undefined; try { inputTexture = generateTexture( device, "r32float", 3, 3, data, GPUTextureUsage.TEXTURE_BINDING | GPUTextureUsage.COPY_SRC, "inputTexture" ); } finally { inputTexture?.destroy(); }});
In questo esempio, la funzione generateTexture()
viene utilizzata per creare una texture di 3x3 pixel con un array di dati
Float32Array
. Dopo aver utilizzato la texture, viene distrutta per liberare le risorse.
#Lettura di una texture
Per trasferire il contenuto di una texture dalla GPU alla CPU, è necessario seguire questi passaggi:
- Creare un buffer di staging, configurato per essere mappabile sulla CPU.
const stagingBuffer = device.createBuffer({ size: bytesPerRow * texture.height, usage: GPUBufferUsage.MAP_READ | GPUBufferUsage.COPY_DST});
- Copiare il contenuto della texture nel buffer di staging utilizzando il metodo
copyTextureToBuffer()
.
const encoder: GPUCommandEncoder = device.createCommandEncoder();encoder.copyTextureToBuffer( { texture }, { buffer: stagingBuffer, bytesPerRow }, { width: texture.width, height: texture.height, depthOrArrayLayers: 1 });const commandBuffer = encoder.finish();device.queue.submit([commandBuffer]);
- Mappare il buffer di staging nella RAM per accedervi dalla CPU.
await stagingBuffer.mapAsync(GPUMapMode.READ);const mappedRange: ArrayBuffer = stagingBuffer.getMappedRange();
- Creare una copia dei dati necessari.
// The slice() method of ArrayBuffer instances returns a new ArrayBuffer// whose contents are a copy of this ArrayBuffer's bytes.const mappedRangeDeepCopy = mappedRange.slice(0);
// When called with an ArrayBuffer instance,// a new typed array view is created that views the specified buffer.return new typedArrayConstructor(mappedRangeDeepCopy);
- Annullare la mappatura del buffer.
stagingBuffer.unmap();
- Distruggere il buffer di staging per rilasciare la memoria GPU.
stagingBuffer.destroy();
Anche in questo caso, è opportuno incapsulare l’intero processo all’interno di una funzione di utility riutilizzabile.
import { TEXTURE_FORMAT_INFO, TypedArray } from '@/texture_metadata.ts';
export async function readTextureData( device: GPUDevice, texture: GPUTexture): Promise<TypedArray> { let stagingBuffer: GPUBuffer; try { const { bytesPerTexel, typedArrayConstructor } = TEXTURE_FORMAT_INFO[texture.format]; const bytesPerRow = bytesPerTexel * texture.width;
// 1) Crea un buffer di staging, configurato per essere mappabile sulla CPU stagingBuffer = device.createBuffer({ label: `stagingBuffer(${texture.format})`, size: bytesPerRow * texture.height, usage: GPUBufferUsage.MAP_READ | GPUBufferUsage.COPY_DST });
30 collapsed lines
// 2) Copia il contenuto della texture nel buffer di staging utilizzando // il metodo copyTextureToBuffer() const encoder: GPUCommandEncoder = device.createCommandEncoder( { label: `readTextureData(${texture.format})` } ); encoder.copyTextureToBuffer( { texture }, { buffer: stagingBuffer, bytesPerRow }, { width: texture.width, height: texture.height, depthOrArrayLayers: 1 } ); const commandBuffer = encoder.finish(); device.queue.submit([commandBuffer]);
// 3) Mappa il buffer di staging nella RAM await stagingBuffer.mapAsync(GPUMapMode.READ); const mappedRange: ArrayBuffer = stagingBuffer.getMappedRange();
// 4) Creare una copia dei dati necessari // The slice() method of ArrayBuffer instances returns a new ArrayBuffer // whose contents are a copy of this ArrayBuffer's bytes. const mappedRangeDeepCopy = mappedRange.slice(0);
// When called with an ArrayBuffer instance, // a new typed array view is created that views the specified buffer. return new typedArrayConstructor(mappedRangeDeepCopy); } finally { if (stagingBuffer) { // 5) Annullare la mappatura del buffer stagingBuffer.unmap();
// 6) Distruggere il buffer di staging per rilasciare la memoria GPU stagingBuffer.destroy(); } }}
La funzione readTextureData()
è progettata per leggere i dati da una texture GPU in modo generico e flessibile.
Per garantire una corretta gestione delle risorse, l’intera logica della funzione è racchiusa in un blocco try-finally
.
Questo approccio assicura che operazioni fondamentali, come l’annullamento della mappatura (unmap()
) e la distruzione del
buffer di staging (destroy()
), vengano eseguite anche in caso di errori.
Nei test può essere utilizzata in questo modo:
test("Create and read textures", async (assert) => { const data = new Float32Array( range(1, 1 + 64 * 64) );
let inputTexture: GPUTexture | undefined; try { inputTexture = generateTexture( device, "r32float", 64, 64, data, GPUTextureUsage.TEXTURE_BINDING | GPUTextureUsage.COPY_SRC, "inputTexture" );
const gpuData = await readTextureData(device, inputTexture); assert.deepEqual(gpuData, data, "Data is read back"); } finally { inputTexture?.destroy(); }})
#Il problema con copyTextureToBuffer()
Se si tenta di leggere una texture 5x5:
test("r32float texture", async (hooks) => { let inputTexture: GPUTexture; try { const data = new Float32Array( range(1, 1 + 5 * 5) );
const inputTexture = generateTexture( device, "r32float", 5, 5, data, GPUTextureUsage.TEXTURE_BINDING | GPUTextureUsage.COPY_SRC, "inputTexture" );
const gpuData = await readTextureData(device, inputTexture); assert.deepEqual(gpuData, data, "Data is read back"); } finally { inputTexture?.destroy(); }});
Otteniamo questi errori:
bytesPerRow (20) is not a multiple of 256.- While encoding [CommandEncoder "readTextureData(r32float)"].CopyTextureToBuffer([Texture "inputTexture"], [Buffer "stagingBuffer(r32float)"], [Extent3D width:5, height:5, depthOrArrayLayers:1]).- While finishing [CommandEncoder "readTextureData(r32float)"].
[Invalid CommandBuffer from CommandEncoder "readTextureData(r32float)"] is invalid.- While calling [Queue].Submit([[Invalid CommandBuffer from CommandEncoder "readTextureData(r32float)"]])
Per motivi di efficienza, WebGPU richiede che il numero di byte per ciascuna riga sia un multiplo di 256. Per risolvere questo problema, è possibile creare uno staging buffer più grande del necessario, così da rispettare il vincolo.
La funzione pad(v, m)
calcola il più piccolo multiplo di m
maggiore o uguale a v
:
function pad(v: number, m: number) { return Math.ceil(v / m) * m;}
bytesPerRow | pad(bytesPerRow, 256) |
---|---|
20 | 256 |
256 | 256 |
320 | 512 |
Restituire un buffer con padding comporta uno spreco di memoria e complica l’uso di asserzioni come deepEqual()
.
Per ovviare a questo problema, è necessario trasferire i dati dallo staging buffer in un buffer di dimensioni corrette.
La funzione sliceMatrix
riorganizza i dati di una matrice, rimuovendo il padding presente nelle righe.
export function sliceMatrix( matrix: ArrayBuffer, bytesPerRow: number, targetBytesPerRow: number): ArrayBuffer { // Sanity checks if (matrix.byteLength === 0) { throw new Error('Input matrix is empty'); }
if (bytesPerRow <= 0 || targetBytesPerRow <= 0) { throw new Error('Bytes per row must be positive'); }
// Ensure matrix size is evenly divisible by bytes per row if (matrix.byteLength % bytesPerRow !== 0) { throw new Error( `Matrix size (${matrix.byteLength} bytes) is not evenly divisible by bytes per row (${bytesPerRow} bytes)` ); }
// Ensure target bytes per row is not larger than source bytes per row if (targetBytesPerRow > bytesPerRow) { throw new Error( `Target bytes per row (${targetBytesPerRow}) cannot be larger than source bytes per row (${bytesPerRow})` ); }
// Calculate height based on total buffer size and current bytes per row const height = matrix.byteLength / bytesPerRow;
// Create a new buffer with the target bytes per row const slicedMatrix = new ArrayBuffer(height * targetBytesPerRow);
// Create views for the source and destination buffers const sourceView = new Uint8Array(matrix); const destinationView = new Uint8Array(slicedMatrix);
// Iterate through rows and copy the relevant portion for (let row = 0; row < height; row++) { // Source row start and slice const sourceRowStart = row * bytesPerRow; const sourceRowSlice = sourceView.subarray( sourceRowStart, sourceRowStart + targetBytesPerRow );
// Destination row start const destRowStart = row * targetBytesPerRow;
// Copy the slice to the destination destinationView.set(sourceRowSlice, destRowStart); }
return slicedMatrix;}
La versione finale di readTextureData
usa sliceMatrix()
se necessario, garantendo che i dati restituiti abbiano il formato corretto.
async function readTextureData( device: GPUDevice, texture: GPUTexture): Promise<TypedArray> { let stagingBuffer: GPUBuffer | undefined = undefined; try { const formatInfo = TEXTURE_FORMAT_INFO[texture.format]!; const bytesPerTexel = formatInfo.bytesPerTexel; const bytesPerRow = pad(bytesPerTexel * texture.width, 256);
20 collapsed lines
// 1) Crea un buffer di staging, configurato per essere mappabile sulla CPU stagingBuffer = device.createBuffer({ label: `stagingBuffer(${texture.format})`, size: bytesPerRow * texture.height, usage: GPUBufferUsage.MAP_READ | GPUBufferUsage.COPY_DST });
// 2) Copia il contenuto della texture nel buffer di staging utilizzando il metodo copyTextureToBuffer() const encoder: GPUCommandEncoder = device.createCommandEncoder( { label: `readTextureData(${texture.format})` } ); encoder.copyTextureToBuffer( { texture }, { buffer: stagingBuffer, bytesPerRow }, { width: texture.width, height: texture.height, depthOrArrayLayers: 1 } ); const commandBuffer = encoder.finish(); device.queue.submit([commandBuffer]);
// 3) Mappa il buffer di staging nella RAM await stagingBuffer.mapAsync(GPUMapMode.READ); const mappedRange: ArrayBuffer = stagingBuffer.getMappedRange();
// Rimuove il padding se necessario if (bytesPerRow !== bytesPerTexel * texture.width) { return new formatInfo.typedArrayConstructor( sliceMatrix( mappedRange, bytesPerRow, bytesPerTexel * texture.width ) ); }
return new formatInfo.typedArrayConstructor(mappedRange.slice(0)); } finally {7 collapsed lines
if (stagingBuffer) { // 5) Annullare la mappatura del buffer stagingBuffer.unmap();
// 6) Distruggere il buffer di staging per rilasciare la memoria GPU stagingBuffer.destroy(); } }}
#Test della sfocatura gaussiana
Con le utility appena descritte, abbiamo tutti gli strumenti necessari per testare la sfocatura gaussiana.
La suite di test in test/browser/gauss_blur_2d.qunit.ts
utilizza una serie di pattern 5x5 (punto singolo, linea, scacchiera,
gradiente) per verificare sia l’implementazione di base che quella ottimizzata:
module("Gaussian Blur 2D", hooks => { let adapter: GPUAdapter; let device: GPUDevice;
hooks.before(async (assert) => { // Request the GPU adapter adapter = (await navigator.gpu.requestAdapter())!; assert.ok(adapter, "Able to request GPU adapter");
// Request the GPU device device = await adapter.requestDevice({ requiredFeatures: [ "timestamp-query", "texture-compression-bc", "float32-filterable" ] }); assert.ok(device, "Able to request GPU device"); });
test("point spread", async (assert) => { const inputData = new Uint8Array([ 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 255, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, ]);
const expectedData = new Uint8Array([ 0, 0, 0, 0, 0, 0, 0, 2, 0, 0, 0, 2, 244, 2, 0, 0, 0, 2, 0, 0, 0, 0, 0, 0, 0, ]);
const expectedData2 = new Uint8Array([ 0, 0, 1, 0, 0, 0, 9, 29, 9, 0, 1, 29, 91, 29, 1, 0, 9, 29, 9, 0, 0, 0, 1, 0, 0, ]);
await basicBlur(assert, "r8uint", inputData, expectedData, 1, 1); await basicBlur(assert, "r8uint", inputData, expectedData2, 2, 1); });
test("point spread float", async (assert) => { const inputData = new Float32Array([ 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, ]);
const v0 = 0.00011810348951257765; const v1 = 0.010631335899233818; const v2 = 0.9570022225379944; const expectedData = new Float32Array([ 0, 0, 0, 0, 0, 0, v0, v1, v0, 0, 0, v1, v2, v1, 0, 0, v0, v1, v0, 0, 0, 0, 0, 0, 0 ]);
await basicBlur(assert, "r32float", inputData, expectedData, 1, 1e-7); });
test("horizontal edge", async (assert) => { const inputData = new Uint8Array([ 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 255, 255, 255, 255, 255, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0, ]);
const expectedData = new Uint8Array([ 0, 0, 0, 0, 0, 2, 2, 2, 2, 2, 246, 249, 249, 249, 246, 2, 2, 2, 2, 2, 0, 0, 0, 0, 0, ]);
await basicBlur(assert, "r8uint", inputData, expectedData, 1, 1); });
test("checkerboard pattern", async (assert) => { const inputData = new Uint8Array([ 255, 0, 255, 0, 255, 0, 255, 0, 255, 0, 255, 0, 255, 0, 255, 0, 255, 0, 255, 0, 255, 0, 255, 0, 255, ]);
const expectedData = new Uint8Array([ 244, 8, 244, 8, 244, 8, 244, 10, 244, 8, 244, 10, 244, 10, 244, 8, 244, 10, 244, 8, 244, 8, 244, 8, 244, ]);
await basicBlur(assert, "r8uint", inputData, expectedData, 1, 1); });
test("gradient", async (assert) => { const inputData = new Uint8Array([ 0, 50, 100, 150, 200, 0, 50, 100, 150, 200, 0, 50, 100, 150, 200, 0, 50, 100, 150, 200, 0, 50, 100, 150, 200 ]);
const expectedData = new Uint8Array([ 0, 49, 98, 148, 195, 0, 50, 100, 150, 197, 0, 50, 100, 150, 197, 0, 50, 100, 150, 197, 0, 49, 98, 148, 195 ]);
await basicBlur(assert, "r8uint", inputData, expectedData, 1, 1); });
async function basicBlur( assert: Assert, format: GPUTextureFormat, inputData: TypedArray, expectedData: TypedArray, kernelRadius: number, tolerance: number ) { // ... }});
I dati attesi per i test sono stati generati con uno script Python basato su Scipy, contenuto in test/resources/gauss_blur.py
.
La funzione basicBlur()
gestisce l’intero processo di test: prima crea una texture di input utilizzando il pattern di test,
poi applica il filtro gaussiano con entrambe le implementazioni, e infine confronta il risultato ottenuto con i valori attesi,
verificando che la differenza rientri nei limiti di tolleranza stabiliti:
async function basicBlur( assert: Assert, format: GPUTextureFormat, inputData: TypedArray, expectedData: TypedArray, kernelRadius: number, tolerance: number) { let inputTexture: GPUTexture | undefined; let blurredTexture: GPUTexture | undefined; let optimizedBlurredTexture: GPUTexture | undefined; try { inputTexture = generateTexture( device, format, 5, 5, inputData, GPUTextureUsage.TEXTURE_BINDING, "inputTexture" );
blurredTexture = await gauss2dBlur( device, inputTexture, kernelRadius );
optimizedBlurredTexture = await gauss2dBlurOptimized( device, inputTexture, kernelRadius );
const blurredTextureData = await readTextureData( device, blurredTexture );
const optimizedBlurredTextureData = await readTextureData( device, optimizedBlurredTexture );
assert.textureMatches( blurredTextureData, expectedData, 5, 5, 1, tolerance, `gauss2dBlur works (k = ${kernelRadius})` );
assert.textureMatches( optimizedBlurredTextureData, expectedData, 5, 5, 1, tolerance, `gauss2dBlurOptimized works (k = ${kernelRadius})` ); } finally { inputTexture?.destroy(); blurredTexture?.destroy(); optimizedBlurredTexture?.destroy(); }}
Questo approccio consente di testare in modo efficace la correttezza delle implementazioni, evitando di duplicare codice tra i diversi casi di test.
Il confronto con i dati delle texture viene effettuato usando il metodo textureMatches()
, che è un’estensione personalizzata
dell’oggetto assert
. Rispetto alla funzione built-in deepEquals()
, textureMatches()
gestisce il confronto con un margine
di errore, che è utile quando si lavora con dati numerici in virgola mobile. Inoltre, questo metodo migliora i messaggi di errore in caso di mismatch,
formattando le matrici in modo più leggibile con il metodo formatMatrix()
:
import { TypedArray } from "@/webgpu/texture_metadata.ts";import { formatMatrix } from "@/utils/math.ts";
QUnit.assert.textureMatches = function( actual: TypedArray, expected: TypedArray, width: number, height: number, channelCount: number = 1, tolerance: number = 0, message?: string) { // Verify the result length matches the expected size const expectedSize = width * height * channelCount; const lengthMatches = actual.length === expectedSize; let mismatchIndex = -1; if (lengthMatches) { mismatchIndex = actual.findIndex( (value, index) => Math.abs(value - expected[index]) > tolerance ); }
const textureMatches = lengthMatches && mismatchIndex === -1;
let actualMsg: string, expectedMsg: string; if (width * height <= 100) { actualMsg = formatMatrix(actual, channelCount, width, height, '\t'); expectedMsg = formatMatrix(expected, channelCount, width, height, '\t'); } else if (mismatchIndex !== -1) { actualMsg = `length: ${actual.length}, actual[${mismatchIndex}] = ${actual[mismatchIndex]}`; expectedMsg = `length: ${expected.length}, expected[${mismatchIndex}] = ${expected[mismatchIndex]}`; } else { actualMsg = `length: ${actual.length}`; expectedMsg = `length: ${expected.length}`; }
this.pushResult({ result: textureMatches, actual: actualMsg, expected: expectedMsg, message: message || `Texture data matches` });}
Per la tipizzazione, è sufficiente estendere l’interfaccia Assert
in un file .d.ts
:
/// <reference types="vite/client" />/// <reference types="qunit" />declare global { interface Assert { textureMatches( actual: TypedArray, expected: TypedArray, width: number, height: number, channelCount: number, tolerance: number, message?: string ): void; }}
export {};
È importante includere questo file nel main principale, insieme agli import degli altri test, come mostrato nell’esempio seguente:
import { test, module } from "qunit";import "@test/browser/filter/gauss_blur_2d.qunit.ts";import "@test/browser/webgpu/read_texture.qunit.ts";import "@test/browser/utils/texture_assert.ts";import '@test/browser/qunit.css';
module("WebGPU", hooks => { test("is available", async (assert) => { assert.ok(navigator.gpu, "WebGPU is supported");
const adapter = await navigator.gpu.requestAdapter(); assert.ok(adapter, "Able to request GPU adapter");
assert.ok(true, `Vendor: ${adapter!.info.vendor}`); assert.ok(true, `Device: ${adapter!.info.device}`); assert.ok(true, `Description: ${adapter!.info.description}`); assert.ok(true, `Architecture: ${adapter!.info.architecture}`); });});
In questo modo, il file con l’estensione dell’interfaccia Assert
viene correttamente incluso e reso disponibile per
l’uso nelle funzioni di test.
#Conclusione
In questo articolo abbiamo visto come testare il codice WebGPU sul browser usando il framework QUnit.
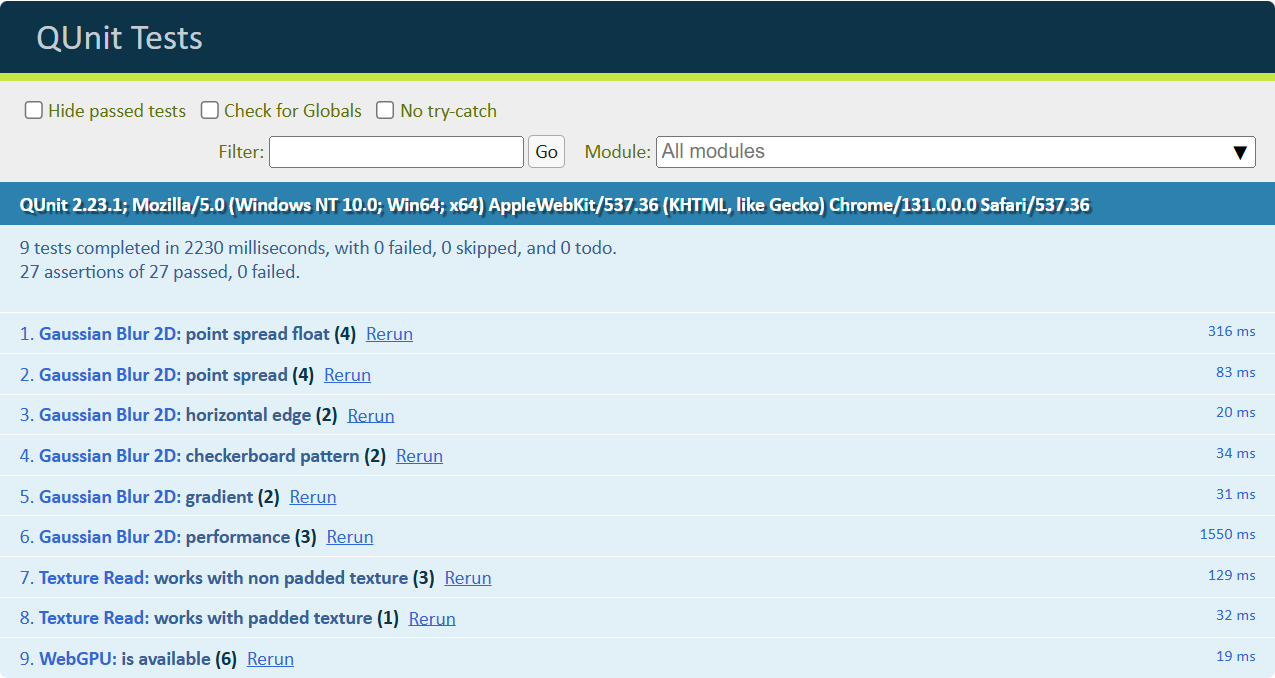
I test completi.
Le funzioni di utility discusse sono particolarmente utili quando si desidera testare codice basato su WebGPU che fa uso delle texture.